(一)从底层透析文件上传的实现,此时并没有介入struts2
1、upload.jsp,在form中属性method默认为get,涉及文件上传时必须改为post,默认
enctype="application/x-www-form-urlencoded" ,我们暂且不修改,看会有什么结果
1
<%
@ page language="java" contentType="text/html; charset=GBK"
2
pageEncoding="GBK"%>
3
<!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd">
4
<html>
5
<head>
6
<meta http-equiv="Content-Type" content="text/html; charset=GB18030">
7
<title>Insert title here</title>
8
</head>
9
<body>
10
<form action="result.jsp" method="post"
11
enctype="application/x-www-form-urlencoded">
12
Information:
13
<input type="text" name="info">
14
<br>
15
File:
16
<input type="file" name="file">
17
<br>
18
<input type="submit" name="submit" value=" submit ">
19
</form>
20
</body>
21
</html>
result.jsp
1
<%
@ page language="java" contentType="text/html; charset=GBK"
2
pageEncoding="GBK"%>
3
<!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd">
4
<html>
5
<head>
6
<meta http-equiv="Content-Type" content="text/html; charset=GB18030">
7
<title>Insert title here</title>
8
</head>
9
<body>
10
Information:<%=request.getParameter("info")%><br>
11
File:<%=request.getParameter("file")%><br>
12
</body>
13
</html>
结果:


2、修改result.jsp页面代码,输出读入的流

<%
@ page import="java.io.*"%>
<body>

<%
InputStream is = request.getInputStream();
BufferedReader br = new BufferedReader(new InputStreamReader(is));
String buffer = null;
while ((buffer = br.readLine()) != null) {
out.print(buffer + "<br>");
}
%>
</body>
结果:

这个结果可以断定,文件的上传并没有成功,而仅仅是上传了文件的路径信息而已
3、把upload.jsp中form的enctype属性改为
enctype="multipart/form-data"
<form action="result.jsp" method="post" enctype="multipart/form-data">
Information:
<input type="text" name="info">
<br>
File:
<input type="file" name="file">
<br>
<input type="submit" name="submit" value=" submit ">
</form>
结果:
说明文件上传是成功的。
(二)手动采用fileupload组建进行文件上传
upload2.jsp
1
<%
@ page language="java" contentType="text/html; charset=GB18030"
2
pageEncoding="GB18030"%>
3
<!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd">
4
<html>
5
<head>
6
<meta http-equiv="Content-Type" content="text/html; charset=GB18030">
7
<title>Insert title here</title>
8
</head>
9
<body>
10
<form action="/MyStruts2/UploadServlet" method="post" enctype="multipart/form-data">
11
username:
12
<input type="text" name="username">
13
<br>
14
password:
15
<input type="password" name="password">
16
<br>
17
file1:
18
<input type="file" name="file1">
19
<br>
20
file2:
21
<input type="file" name="file2">
22
<br>
23
<input type="submit" value=" submit ">
24
</form>
25
</body>
26
</html>
web.xml中的配置
<servlet>
<servlet-name>UploadServlet</servlet-name>
<servlet-class>com.test.servlet.UploadServlet</servlet-class>
</servlet>

<servlet-mapping>
<servlet-name>UploadServlet</servlet-name>
<url-pattern>/UploadServlet</url-pattern>
</servlet-mapping>
UploadServle.java
1
package com.test.servlet;
2
3
import java.io.File;
4
import java.io.FileOutputStream;
5
import java.io.IOException;
6
import java.io.InputStream;
7
import java.io.OutputStream;
8
import java.util.List;
9
10
import javax.servlet.ServletException;
11
import javax.servlet.http.HttpServlet;
12
import javax.servlet.http.HttpServletRequest;
13
import javax.servlet.http.HttpServletResponse;
14
15
import org.apache.commons.fileupload.FileItem;
16
import org.apache.commons.fileupload.FileUploadException;
17
import org.apache.commons.fileupload.disk.DiskFileItemFactory;
18
import org.apache.commons.fileupload.servlet.ServletFileUpload;
19
20
@SuppressWarnings("serial")
21
public class UploadServlet extends HttpServlet
{
22
@SuppressWarnings(
{ "unchecked", "deprecation" })
23
public void doPost(HttpServletRequest request, HttpServletResponse response)
24
throws ServletException, IOException
{
25
26
DiskFileItemFactory factory = new DiskFileItemFactory();
27
28
String path = request.getRealPath("/upload");
29
30
factory.setRepository(new File(path));
31
32
factory.setSizeThreshold(1024 * 1024);
33
34
ServletFileUpload upload = new ServletFileUpload(factory);
35
36
try
{
37
List<FileItem> list = upload.parseRequest(request);
38
39
for (FileItem item : list)
{
40
if (item.isFormField())
{
41
String name = item.getFieldName();
42
43
String value = item.getString("gbk");
44
45
System.out.println(name);
46
47
request.setAttribute(name, value);
48
} else
{
49
String name = item.getFieldName();
50
51
String value = item.getName();
52
53
int start = value.lastIndexOf("\\");
54
55
String fileName = value.substring(start + 1);
56
57
request.setAttribute(name, fileName);
58
59
item.write(new File(path, fileName));
60
61
OutputStream os = new FileOutputStream(new File(path,
62
fileName));
63
64
InputStream is = item.getInputStream();
65
66
byte[] buffer = new byte[400];
67
68
int length = 0;
69
70
while ((length = is.read(buffer)) > 0)
{
71
os.write(buffer, 0, length);
72
}
73
74
os.close();
75
76
is.close();
77
78
}
79
}
80
}
81
82
catch (Exception ex)
{
83
ex.printStackTrace();
84
}
85
request.getRequestDispatcher("upload/result2.jsp").forward(request,
86
response);
87
}
88
89
}
结果:
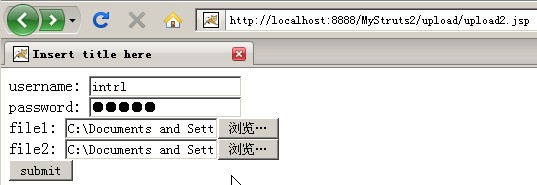
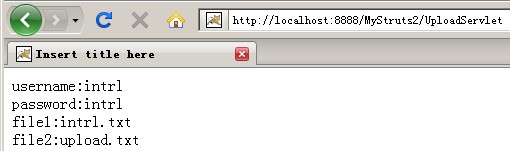
(三)使用struts2进行文件上传、下载
需引入两个jar包
commons-fileupload-1.2.1.jar
commons-io-1.3.2.jar
这两个jar包在struts2.1.6版本中已经自带,较低版本需到apache网站下载,网址:
http://commons.apache.org/1、单文件上传
upload3.jsp
1
<%
@ page language="java" contentType="text/html; charset=GB18030"
2
pageEncoding="GB18030"%>
3
<%
@ taglib prefix="s" uri="/struts-tags"%>
4
<!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd">
5
<html>
6
<head>
7
<meta http-equiv="Content-Type" content="text/html; charset=GB18030">
8
<title>Insert title here</title>
9
</head>
10
<body>
11
<s:form action="upload" method="post" theme="simple"
12
enctype="multipart/form-data">
13
<table align="center" width="50%" border="1">
14
<tr>
15
<td>
16
username
17
</td>
18
<td>
19
<s:textfield name="username"></s:textfield>
20
</td>
21
</tr>
22
<tr>
23
<td>
24
password
25
</td>
26
<td>
27
<s:password name="password"></s:password>
28
</td>
29
</tr>
30
<tr>
31
<td>
32
file
33
</td>
34
35
<td>
36
<s:file name="file"></s:file>
37
38
</td>
39
</tr>
40
<tr>
41
<td>
42
<s:submit value=" submit "></s:submit>
43
</td>
44
<td>
45
<s:reset value=" reset "></s:reset>
46
</td>
47
</tr>
48
</table>
49
</s:form>
50
</body>
51
</html>
web.xml中的配置
<filter>
<filter-name>struts2</filter-name>
<filter-class>
org.apache.struts2.dispatcher.FilterDispatcher
</filter-class>
</filter>

<filter-mapping>
<filter-name>struts2</filter-name>
<url-pattern>/*</url-pattern>
</filter-mapping>
struts.xml中的配置
1
<?xml version="1.0" encoding="GBK" ?>
2
<!DOCTYPE struts PUBLIC
3
"-//Apache Software Foundation//DTD Struts Configuration 2.0//EN"
4
"http://struts.apache.org/dtds/struts-2.0.dtd">
5
6
<struts>
7
<constant name="struts.i18n.encoding" value="gbk"></constant>
8
<constant name="struts.multipart.saveDir" value="c:\"></constant>
9
<package name="struts2" extends="struts-default">
10
<action name="upload" class="com.test.action.UploadAction">
11
<result name="success">/upload/result3.jsp</result>
12
</action>
13
</package>
14
</struts>
UploadAction.java
1
package com.test.action;
2
3
import java.io.File;
4
import java.io.FileInputStream;
5
import java.io.FileOutputStream;
6
import java.io.InputStream;
7
import java.io.OutputStream;
8
9
import org.apache.struts2.ServletActionContext;
10
11
import com.opensymphony.xwork2.ActionSupport;
12
13
@SuppressWarnings("serial")
14
public class UploadAction extends ActionSupport
{
15
private String username;
16
private String password;
17
private File file;
18
private String fileFileName;
19
private String fileContentType;
20
21
public String getUsername()
{
22
return username;
23
}
24
25
public void setUsername(String username)
{
26
this.username = username;
27
}
28
29
public String getPassword()
{
30
return password;
31
}
32
33
public void setPassword(String password)
{
34
this.password = password;
35
}
36
37
public File getFile()
{
38
return file;
39
}
40
41
public void setFile(File file)
{
42
this.file = file;
43
}
44
45
public String getFileFileName()
{
46
return fileFileName;
47
}
48
49
public void setFileFileName(String fileFileName)
{
50
this.fileFileName = fileFileName;
51
}
52
53
public String getFileContentType()
{
54
return fileContentType;
55
}
56
57
public void setFileContentType(String fileContentType)
{
58
this.fileContentType = fileContentType;
59
}
60
61
@SuppressWarnings("deprecation")
62
@Override
63
public String execute() throws Exception
{
64
InputStream is = new FileInputStream(file);
65
String root = ServletActionContext.getRequest().getRealPath("/upload");
66
File destFile = new File(root, this.getFileFileName());
67
OutputStream os = new FileOutputStream(destFile);
68
byte[] buffer = new byte[400];
69
70
int length = 0;
71
72
while ((length = is.read(buffer)) > 0)
{
73
os.write(buffer, 0, length);
74
}
75
is.close();
76
os.close();
77
return SUCCESS;
78
}
79
}
结果:

2、多文件上传
修改action
private List<File> file;
private List<String> fileFileName;
private List<String> fileContentType;

public String execute() throws Exception
{

for (int i = 0; i < file.size(); ++i)
{
InputStream is = new FileInputStream(file.get(i));
String root = ServletActionContext.getRequest().getRealPath(
"/upload");
File destFile = new File(root, this.getFileFileName().get(i));
OutputStream os = new FileOutputStream(destFile);
byte[] buffer = new byte[400];

int length = 0;


while ((length = is.read(buffer)) > 0)
{
os.write(buffer, 0, length);
}
is.close();
os.close();
}
return SUCCESS;
}
修改upload3.jsp
<tr>
<td>
file1
</td>
<td>
<s:file name="file"></s:file>
</td>
</tr>
<tr>
<td>
file2
</td>
<td>
<s:file name="file"></s:file>
</td>
</tr>
<tr>
<td>
file3
</td>
<td>
<s:file name="file"></s:file>
</td>
</tr>
结果:
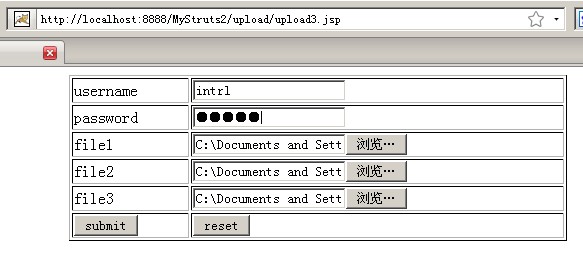

3、任意数量文件上传
在多文件上传的基础上修改upload3.jsp

<script type="text/javascript">
function addMore()

{
var td = document.getElementById("more");
var br = document.createElement("br");
var input = document.createElement("input");
var button = document.createElement("input");
input.type = "file";
input.name = "file";
button.type = "button";
button.value = "Remove";
button.onclick = function()

{
td.removeChild(br);
td.removeChild(input);
td.removeChild(button);
}
td.appendChild(br);
td.appendChild(input);
td.appendChild(button);
}
</script>
<tr>
<td>
file1
</td>
<td id="more">
<s:file name="file"></s:file>
<input type="button" value="Add More.." onclick="addMore()">
</td>
</tr>
结果:
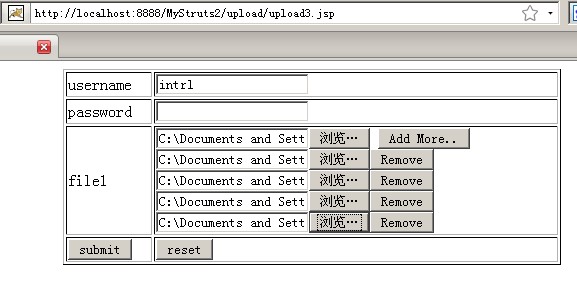
(四)文件上传类型、大小的限制
使用struts的拦截器,struts2-core-2.1.6.jar/org.apache.struts2.interceptor.FileUploadInterceptor.class的源码中我们可以看到:
public class FileUploadInterceptor extends AbstractInterceptor {

private static final long serialVersionUID = -4764627478894962478L;

protected static final Logger LOG = LoggerFactory.getLogger(FileUploadInterceptor.class);
private static final String DEFAULT_MESSAGE = "no.message.found";

protected boolean useActionMessageBundle;

protected Long maximumSize;
protected Set<String> allowedTypesSet = Collections.emptySet();
protected Set<String> allowedExtensionsSet = Collections.emptySet();
所以我们只需的struts.xml中配置它的属性allowedTypesSet即可。在action节点中修改拦截器(默认的拦截器中已经有fileUpload拦截器,我们必须提取出来进行参数设置,然后在加上默认的拦截器)。
<action name="upload" class="com.test.action.UploadAction">
<result name="success">/upload/result3.jsp</result>
<result name="input">/upload/upload3.jsp</result>
<interceptor-ref name="fileUpload">
<param name="maximumSize">409600</param>
<param name="allowedTypes">
application/vnd.ms-powerpoint
</param>
</interceptor-ref>
<interceptor-ref name="defaultStack"></interceptor-ref>
</action>
其中
<param name="allowedTypes">application/vnd.ms-powerpoint</param>的allowedTypes的值可在C:\Tomcat 6.0\conf的web.xml文件中查找。

报错信息:
严重: Content-Type not allowed: file "intrl.txt" "upload__138d8aca_120b73e9cf4__8000_00000002.tmp" text/plain
(五)文件的下载
download.jsp
<s:a href="/MyStruts2/download.action">download</s:a>
DownloadAction.java
1
package com.test.action;
2
3
import java.io.InputStream;
4
5
import org.apache.struts2.ServletActionContext;
6
7
import com.opensymphony.xwork2.ActionSupport;
8
9
public class DownloadAction extends ActionSupport
{
10
public InputStream getDownloadFile()
{
11
return ServletActionContext.getServletContext().getResourceAsStream(
12
"/upload/intrl.ppt");
13
}
14
15
@Override
16
public String execute() throws Exception
{
17
return SUCCESS;
18
}
19
}
20
web.xml中action配置
<action name="download"
class="com.test.action.DownloadAction">
<result name="success" type="stream">
<param name="contentType">
application/vnd.ms-powerpoint
</param>
<param name="contentDisposition">
filename="intrl.ppt"
</param>
<param name="inputName">downloadFile</param>
</result>
</action>
结果:
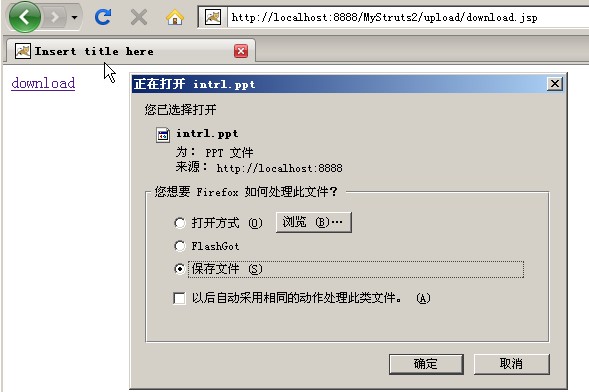