提供了FlowLayout、BorderLayout、GriderLayout、GridBagLayout、CardLayout五个常用的布局管理器,Swing还提供了一个BoxLayout布局管理器
1
1/** *//**
2
* @(#)TestLayout.java
3
*
4
*
5
* @author 叶云策(intrl)
6
* @version 1.00 2009/5/17
7
*/
8
9
import java.awt.*;
10
import java.awt.event.*;
11
import javax.swing.BoxLayout;
12
import javax.swing.Box;
13
public class TestLayout
14

{
15
public static void main(String[] args)
16
{
17
//FlowLayout布局管理
18
TestFlowLayout tfl=new TestFlowLayout();
19
tfl.init();
20
21
//BorderLayout布局管理
22
new TestBorderLayout().init();
23
24
//GridLayout布局管理
25
new TestGridLayout().init();
26
27
//GridBagLayout布局管理
28
new TestGridBagLayout().init();
29
30
//CardLayout布局管理
31
new TestCardLayout().init();
32
33
//绝对定位
34
new TestNullLayout().init();
35
36
//BoxLayout布局管理
37
new TextBoxLayout1().init();
38
new TextBoxLayout2().init();
39
new TextBoxLayout3().init();
40
41
}
42
}
43
//FlowLayout布局管理
44
class TestFlowLayout
45

{
46
private Frame f=new Frame("FlowLayout布局管理");
47
public void init()
48
{
49
f.setLayout(new FlowLayout(FlowLayout.LEFT,20,5));
50
for(int i=0;i<10;i++)
51
{
52
f.add(new Button("按钮"+i));
53
}
54
f.pack();
55
f.setVisible(true);
56
}
57
}
58
//BorderLayout布局管理
59
class TestBorderLayout
60

{
61
private Frame f=new Frame("BorderLayout布局管理");
62
Panel p=new Panel();
63
public void init()
64
{
65
f.setLayout(new BorderLayout(30,5));
66
f.add(new Button("南"),BorderLayout.SOUTH);
67
f.add(new Button("北"),BorderLayout.NORTH);
68
p.add(new TextField(20));
69
p.add(new Button("单击我"));
70
f.add(p);
71
f.add(new Button("东"),BorderLayout.EAST);
72
f.pack();
73
f.setVisible(true);
74
}
75
}
76
//GridLayout布局管理
77
class TestGridLayout
78

{
79
private Frame f=new Frame("GridLayout布局管理");
80
Panel p1=new Panel();
81
Panel p2=new Panel();
82
public void init()
83
{
84
p1.add(new TextField(30));
85
f.add(p1,BorderLayout.NORTH);
86
p2.setLayout(new GridLayout(3,5,4,4));
87
String[] name=
{"0","1","2","3","4","5","6","7","8","9","+","-","*","/","."};
88
for(int i=0;i<name.length;i++)
89
{
90
p2.add(new Button(name[i]));
91
}
92
f.add(p2);
93
f.pack();
94
f.setVisible(true);
95
}
96
}
97
//GridBagLayout布局管理
98
class TestGridBagLayout
99

{
100
private Frame f=new Frame("GridBagLayout布局管理");
101
private GridBagLayout gb=new GridBagLayout();
102
private GridBagConstraints gbc=new GridBagConstraints();
103
private Button[] bs=new Button[10];
104
public void init()
105
{
106
f.setLayout(gb);
107
for(int i=0;i<bs.length;i++)
108
{
109
bs[i]=new Button("按钮"+i);
110
}
111
//所有组件都可以横向、纵向上扩大
112
gbc.fill=GridBagConstraints.BOTH;
113
gbc.weightx=1;
114
addButton(bs[0]);
115
addButton(bs[1]);
116
addButton(bs[2]);
117
//该GridBagConstraints控制的GUI组件将会成为横向最后一个元素
118
gbc.gridwidth=GridBagConstraints.REMAINDER;
119
addButton(bs[3]);
120
//该GridBagConstraints控制的GUI组件将横向上不会扩大
121
gbc.weightx=0;
122
addButton(bs[4]);
123
//该GridBagConstraints控制的GUI组件将横跨两个网络
124
gbc.gridwidth=2;
125
addButton(bs[5]);
126
//该GridBagConstraints控制的GUI组件将横跨一个网络
127
gbc.gridwidth=1;
128
//该GridBagConstraints控制的GUI组件将纵向跨两个网络
129
gbc.gridheight=2;
130
//该GridBagConstraints控制的GUI组件将会成为横向最后一个元素
131
gbc.gridwidth=GridBagConstraints.REMAINDER;
132
addButton(bs[6]);
133
//该GridBagConstraints控制的GUI组件将横向跨一个网络,纵向跨两个网络
134
gbc.gridwidth=1;
135
gbc.gridheight=2;
136
//该GridBagConstraints控制的GUI组件纵向扩大的权重是1
137
gbc.weighty=1;
138
addButton(bs[7]);
139
//设置下面的按钮在纵向上不会扩大
140
gbc.weighty=0;
141
//该GridBagConstraints控制的GUI组件将会成为横向最后一个元素
142
gbc.gridwidth=GridBagConstraints.REMAINDER;
143
//该GridBagConstraints控制的GUI组件将纵向跨一个网络
144
gbc.gridheight=1;
145
addButton(bs[8]);
146
addButton(bs[9]);
147
f.pack();
148
f.setVisible(true);
149
}
150
private void addButton(Button button)
151
{
152
gb.setConstraints(button,gbc);
153
f.add(button);
154
}
155
}
156
//CardLayout布局管理
157
class TestCardLayout
158

{
159
private Frame f=new Frame("TestCardLayout布局管理器");
160
private String[] names=
{"第一张","第二张","第三张","第四张","第五张"};
161
private Panel pl=new Panel();
162
private CardLayout c=new CardLayout();
163
public void init()
164
{
165
pl.setLayout(c);
166
for(int i=0;i<names.length;i++)
167
{
168
pl.add(names[i],new Button(names[i]));
169
}
170
Panel p=new Panel();
171
172
Button previous=new Button("上一张");
173
previous.addActionListener(new ActionListener()
{
174
public void actionPerformed(ActionEvent e)
175
{
176
c.previous(pl);
177
}
178
});
179
180
Button next=new Button("下一张");
181
next.addActionListener(new ActionListener()
{
182
public void actionPerformed(ActionEvent e)
183
{
184
c.next(pl);
185
}
186
});
187
188
Button first=new Button("第一张");
189
first.addActionListener(new ActionListener()
{
190
public void actionPerformed(ActionEvent e)
191
{
192
c.first(pl);
193
}
194
});
195
196
Button last=new Button("最后一张");
197
last.addActionListener(new ActionListener()
{
198
public void actionPerformed(ActionEvent e)
199
{
200
c.last(pl);
201
}
202
});
203
204
Button third=new Button("第三张");
205
third.addActionListener(new ActionListener()
{
206
public void actionPerformed(ActionEvent e)
207
{
208
c.show(pl,"第三张");
209
}
210
});
211
212
p.add(previous);
213
p.add(next);
214
p.add(first);
215
p.add(last);
216
p.add(third);
217
f.add(pl);
218
f.add(p,BorderLayout.SOUTH);
219
f.pack();
220
f.setVisible(true);
221
}
222
}
223
//绝对定位(它不是最好的方法,可能导致该GUI界面失去跨平台特性)
224
class TestNullLayout
225

{
226
Frame f=new Frame("绝对定位");
227
Button b1=new Button("第一个按钮");
228
Button b2=new Button("第二个按钮");
229
public void init()
230
{
231
f.setLayout(null);
232
b1.setBounds(20,30,90,28);
233
f.add(b1);
234
b2.setBounds(50,45,120,35);
235
f.add(b2);
236
f.setBounds(50,50,200,200);
237
f.setVisible(true);
238
}
239
}
240
//BoxLayout布局管理
241
class TextBoxLayout1
242

{
243
private Frame f=new Frame("BoxLayout布局管理1");
244
public void init()
245
{
246
f.setLayout(new BoxLayout(f,BoxLayout.Y_AXIS));
247
//下面按钮将会垂直排列
248
f.add(new Button("第一个按钮"));
249
f.add(new Button("按钮二"));
250
f.pack();
251
f.setVisible(true);
252
}
253
}
254
//BoxLayout布局管理
255
class TextBoxLayout2
256

{
257
private Frame f=new Frame("BoxLayout布局管理2");
258
private Box horizontal=Box.createHorizontalBox();
259
private Box vertical=Box.createVerticalBox();
260
public void init()
261
{
262
horizontal.add(new Button("水平按钮一"));
263
horizontal.add(new Button("水平按钮二"));
264
vertical.add(new Button("垂直按钮一"));
265
vertical.add(new Button("垂直按钮二"));
266
f.add(horizontal,BorderLayout.NORTH);
267
f.add(vertical);
268
f.pack();
269
f.setVisible(true);
270
}
271
}
272
//BoxLayout布局管理
273
class TextBoxLayout3
274

{
275
private Frame f=new Frame("BoxLayout布局管理3");
276
private Box horizontal=Box.createHorizontalBox();
277
private Box vertical=Box.createVerticalBox();
278
public void init()
279
{
280
horizontal.add(new Button("水平按钮一"));
281
horizontal.add(Box.createHorizontalGlue());
282
horizontal.add(new Button("水平按钮二"));
283
//水平方向不可拉伸的间距,其宽度为10px
284
horizontal.add(Box.createHorizontalStrut(10));
285
horizontal.add(new Button("水平按钮三"));
286
vertical.add(new Button("垂直按钮一"));
287
vertical.add(Box.createVerticalGlue());
288
vertical.add(new Button("垂直按钮二"));
289
//垂直方向不可拉伸的间距,其高度为10px
290
vertical.add(Box.createVerticalStrut(10));
291
vertical.add(new Button("垂直按钮三"));
292
f.add(horizontal,BorderLayout.NORTH);
293
f.add(vertical);
294
f.pack();
295
f.setVisible(true);
296
}
297
}
运行结果截图:
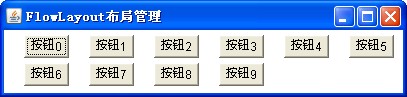
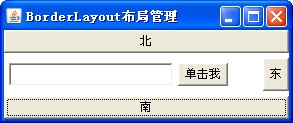
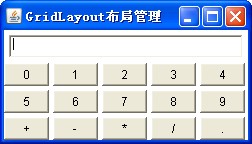


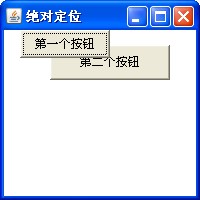


源代码下载:
/Files/intrl/TestLayout.rar